How to convert List to DataTable - C#,ASP.NET
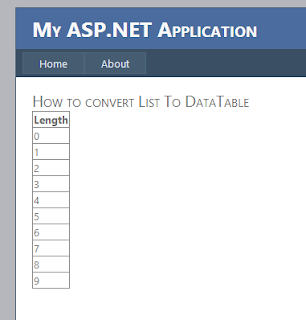
Short Description This example will show how to convert List<T> where T could be any object and convert the List<T> to DataTable. The example will use two class which is System.ComponentModel; and System.Data; Note : The Convertion class extention method must be in static because System.ComponentModel.TypeDescriptor is a static method In this example i will use asp.net . The ASPX page <h1> How to convert List To DataTable </h1> <asp:GridView ID="GridView1" runat="server"> </asp:GridView> The Code Behind protected void Page_Load(object sender, EventArgs e) { List<string> listOfString = new List<string>(); ...